Javascript Performance and Snippets part 2
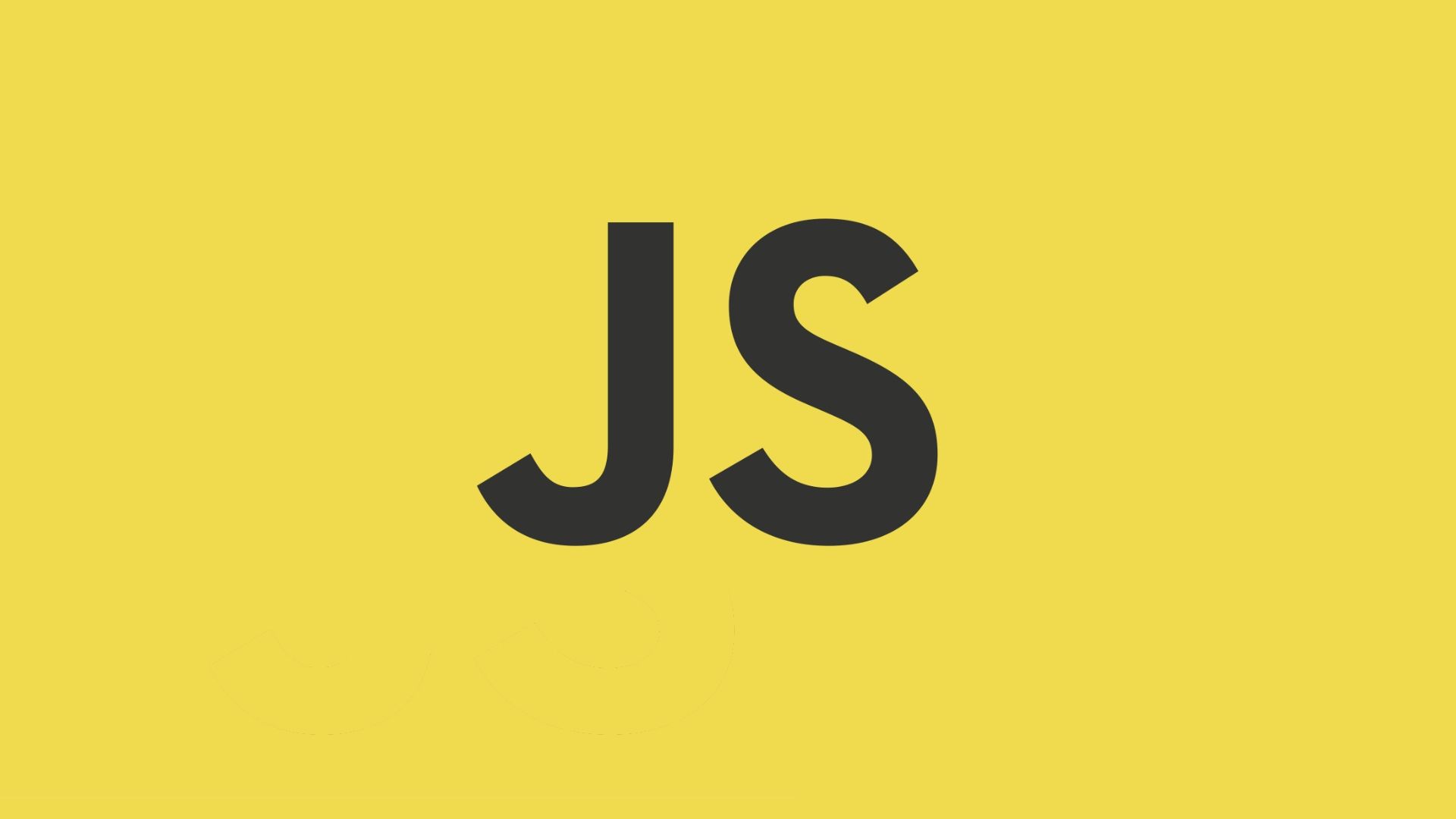
I have a bit more time playing around with JavaScript and I decided that it is time to post another JavaScript tips and tricks/gotchas. In today's blog I will be going over some of efficiencies and draw backs of using libraries that I have become aware of. Also I will be posting some of the gotchas that you may or may not have know with JavaScript. :D Alright lets get going!

Jsperf.com
Over the past few months I was given a challenge of finding inefficiencies with our code. It was a pretty daunting task as I (being a new developer) was going to look over code of higher skilled people and see if I can find where the code is chugging. At first I didn't know where to start, and even after I started I didn't find anything that really did much performance wise. Then I found jsperf.com.
Jsperf uses benchmark.js so that you can compare libraries and other JavaScript functions to see which one is the fastest and what browser performs the best. Little did I know that it was going to reveal some light on how fast IE is with JavaScript compared to other browsers. What I mean by fast is: Slower than dirt... Sometimes.
Add/Remove Classes
First off let me start with adding and removing classes from an element using various ways of doing that.
This one primarily points out that maybe using vanilla would be a better approach to this problem. In chrome it would seem it is super good at doing this, where as IE11 it is quite slow and Firefox isn't too far ahead of IE.
Property Checking
This next one will show how godly good IE is at setting properties.
As you can see here, for some reason IE is really good at doing this, but Chrome and Firefox is not.
Property Checking
This next one is checking properties on elements.
As you can see with this one, Firefox super excels at this, but IE and mobile Chrome is terrible at doing this sort of stuff.
JsFiddle Examples
Now moving onto some of the gotchas and tips/tricks with Javascript.
Events
Events are pretty cool! I started messing around with Socket.io in node.js. If you haven't done that yet, it is definitely something that should be on your to-do list.
Here I was mostly playing around with passing around data with the event to see if I could make it simple to do. It isn't too complex but it was interesting to do regardless.
Comparators
This one has a lot of craziness. This is why we want to use ===
instead of ==
. The only times that I have ever used ==
was when I needed to check to see if a value really equaled null
or undefined
.
==
can be pretty useful if you know what it is doing. As you can see with this fiddle, it can check if "true"
is equal to 1
. ==
will do a type conversion before it does the comparison so that is why ===
will return different results.
In JavaScript the Good Parts
it is recommended to always use ===
just because it is a little more sensitive and will produce an absolute true and false result. If they are the same object and reference in memory, it will return true. Check it out!
Truthy Falsey
This one kind of ties into the previous fiddle; here I am showing how objects will react to if statements. Do they return true or false if you run them through an if check. Some of them might surprise you.
Conclusion
As always I hope this was informative. These are just some of the things that I have been discovering at work. I have found great fun out of doing these tests and think they are great for keeping as a reminder. Until next time.