Passing variables from the server to Browser
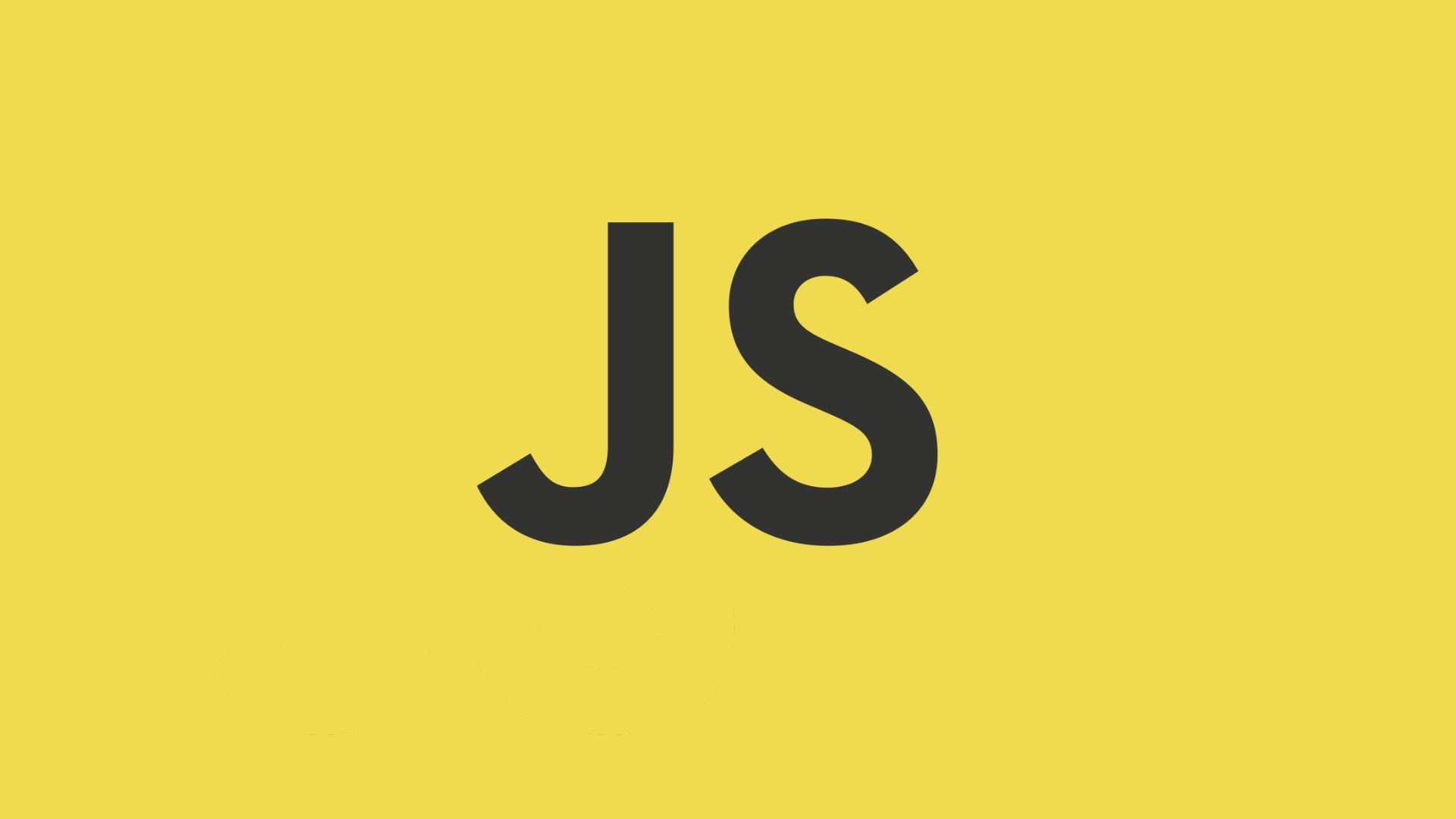
Recently I have been struggling with trying to figure out a good practice for sending information to the view from the server. The main issue I had with this was that I couldn't seem to figure out a good practice for sending some information down without it being exposed on the DOM in some fashion. Now once it is on the client the client can access it if they know how. But there doesn't really seem to be a good practice from what I can tell.
Some of the practices I have seen are:
ViewModel
Sending data down through a view model. The view engine we use ends up parsing that down to a string and placing it somewhere on the DOM. You could put it in a hidden text field (which is probably the most common on the web) or you could just place it in some tag. I hate this approach. It doesn't make any sense. I personally don't want to place my endpoint strings on a searchable platform. I would like to keep them as discreet as I can.
// MyModelViewModel.cs
public class MyModelViewModel{
public string Name {get; set;}
}
<!--index.spark-->
<input type="text" value="@Model"/>
<!--... Converts to-->
<input type="text" value="{Model: {Name: \"Hello\"}}"/>
Can you imagine if this was a large object with a ton of information? One of the things we do for work is create things called tokens. These tokens help with locality support and when we push this information down it gets appended to a init function so Angular can use it. Its HUGE and very ugly on the DOM element.
Ajax
The other approach I have seen is just querying them in an Ajax call when the page loads. This is nice, except now I have a single endpoint that I am calling that grabs all my variables that anyone else could access. Granted I could put some kind of validation token in my request that the server could validate against.
$.ajax("/some-path",{
success: function(data){
yay = data.myData;
}
});
File
Another approach would be sending it down as a Javascript file on the request. This would give you access to the information on load. This is probably a little closer to what I am asking for, but it feels very disconnected from the flow of how you would program the web.
// myModelInfo.js
MyModel = function(){
return {something: "hello"};
};
<!--index.html-->
<script type="text/javascript" src="../src/myModelInfo.js"/>
My issues
My main argument is why isn't there at least some option of an (enter any compiled language name here) objects being directly passed down as a Javascript object to the view on client request. To me it would seem like that should be a thing. Some people that I have discussed this with have said it must not be a big enough deal to implement such a feature. What I say about that is, if there is a pattern of shoving this content into a hidden input field on the DOM so that you can later access it; I feel there is some kind of need. You also wouldn't need to query the DOM to get the content out of a field if it was just given to you on the response.
If there was a way for the server to send down a particular kind of response to which the browser would know how to handle and set up Javascript objects. Something like this:
Model.myViewModel
Am I venturing too far away from base? Am I making things too complex? Are there solutions out there that solve this problem without exposing endpoints or pushing things to the DOM?